Automating add-in installation
To provide users of the RingCentral desktop app with the best possible experience to install your app, developers can optionally implement a web-based user interface that facilitates the installation process. This often includes the following:
- Authenticate the user with the target service
- Allow the user to elect what notifications they would like to subscribe to
- Install the generated webhook URL into the target service
Third-party service prerequisites
To create a simple form to automate the setup of an add-in, the target third-party service in question must support the following:
- OAuth for user authentication and authorization.
- An API for creating, updating and deleting webhooks.
Providing an fully-integrated experience
The installation process you implement will be delivered as a simple web app, and will be rendered to the user during the installation flow via an iframe.
iFrame dimensions
Width | Height |
---|---|
570px | 260px |
Design and Development Guidelines
To accomplish the above, developers need to create what amounts to a mini-web application. This web app will then be embedded into the install flow via an iFrame. We recommend developers adhere to the following guidelines when building this app.
-
Present only a simple form. Try to minimize or suppress entirely branding and other UI-elements that might distract the user from completing the installation process.
-
Do not display a submit button. The submission of your form will be triggered via a javascript callback.
-
Limit your app to a single screen. Other than the step in which you present the user with a button to login, limit your setup form to a single page.
Installation user and developer flow
Team and chat selection
The first step of the installation flow is controlled entirely by RingCentral and allows the user performing the installation to select the chat into which the app will be installed.
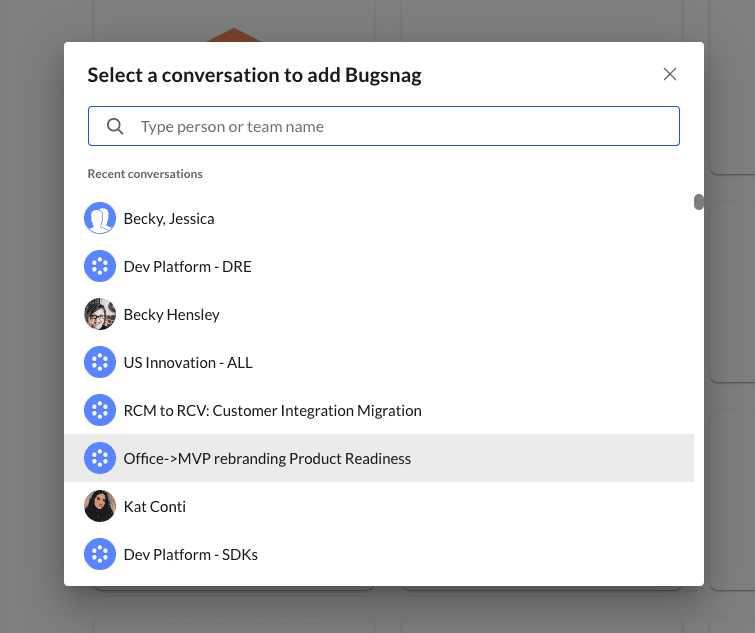
Incoming webhook URL generation
Once the user has selected to chat into which they want the app installed, RingCentral will generate an incoming webhook associated with that chat. The generated URL will be transmitted to the application in the next step.
Load and display iframe
In the next step, RingCentral will render an iframe (570x260 pixels) loading the URL specified by the developer when the application was created. Passed to this URL will be a query parameter called webhook
whose value will be the incoming webhook URL generated by RingCentral in the previous step.
Example iframe
<iframe height="260" width="570"
src="https://example.com/path/to/app?webhook=https://hooks.glip.com/webhook/v2/gdhs7828ergdj72">
</iframe>
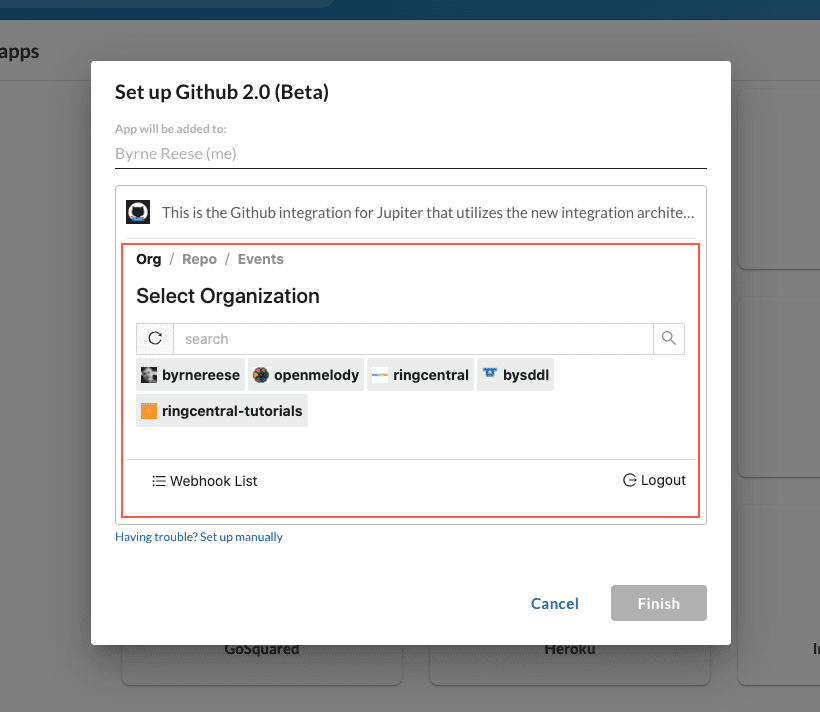
Lookup webhook URL locally
When an iframe is loaded, the developer must disambiguate between a user who is installing a new app, or editing an existing one. The best way to do this is to lookup the webhook URL passed to the service via the iframe in a local datastore. If the webhook URL has been installed previously, then the application should in subsequent steps update the existing webhook. If this is the first time this service is encountering the webhook, then it is safe to proceed to install the webhook.
Storing the webhook locally
In the subsequent step entitled "Store access token" we recommend that developers store in their database a record of every webhook URL that is installed via this process. Associated with each webhook URL developers can also store:
- The access token generated through the OAuth flow.
- The identity of the user installing the application.
- Any subscription preferences associated with the webhook URL.
Authenticate user
Inside the iframe the user will first need to establish their identity by authenticating themselves via the OAuth protocol.
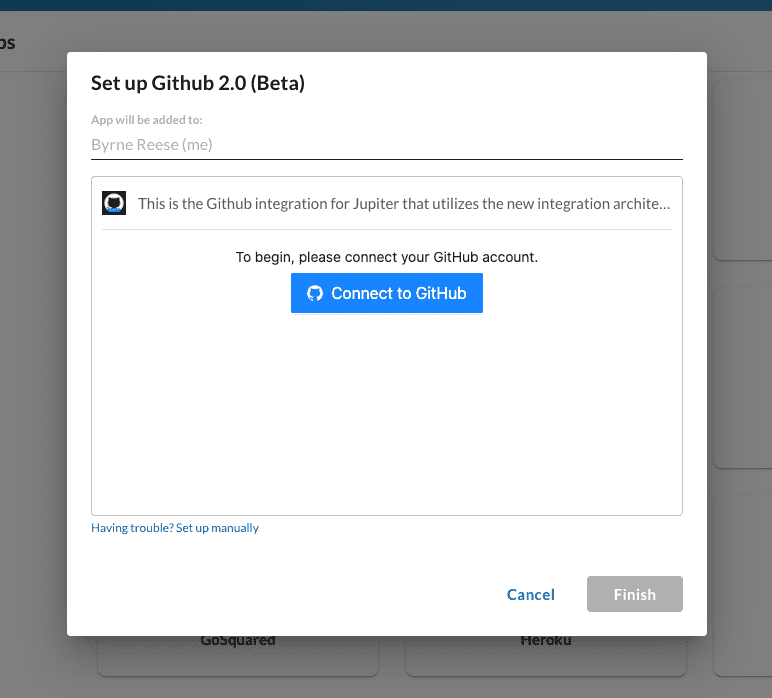
The OAuth process can be launched through a javascript command facilitated by a helper library created by RingCentral.
import { RingCentralNotificationIntegrationHelper } from 'ringcentral-notification-integration-helper'
const app = new RingCentralNotificationIntegrationHelper()
// Open window with proper params so user can do authorization
// in opened window by RingCentral
app.openWindow(windowUrl: string)
Check out the the Add-in App Helper on Github
The RingCentral Add-in App Helper is a Javascript library to help developers implement the necessary Javascript controls to manage their installation app.
Store access token
It is recommended that the application store the access token generated through the OAuth flow and associate that access token with the incoing webhook passed to the service via the iframe. This will allow users to more easily edit the application after it is installed, as the underlying service will be able to more easily lookup the webhook URL, and reconnect the service in which the URL is ultimately installed.
Select subscription preferences
It is common for applications to present users with the option to select what events specifically they would like to subscribe to. This is often required in order to designate how and when the incoming webhook URL will be triggered.
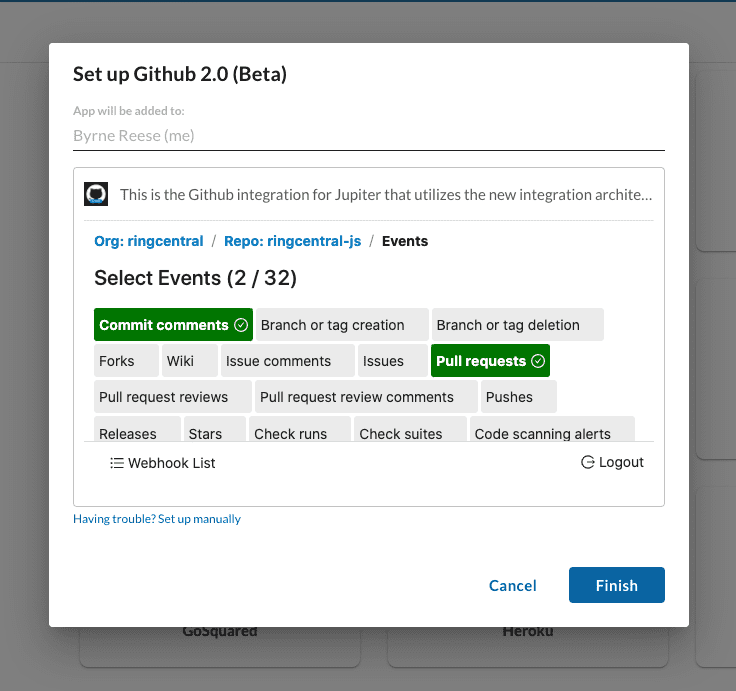
Take for example an add-in being created for a service like Github in which a user would need to first select a repository before setting up a new webhook integration. Then upon selecting a repository, the user would then elect which events (e.g. a pull request, a new comment, a commit, a comment, etc.) associated with that repository they would like to receive notifications.
Install incoming webhook URL
To complete the installation, the developer will instruct the target service, e.g. Jira in the example above, to send webhooks to the incoming webhook URL in response to the events selected in the previous step.
Enable the install dialog's "Finish" button
When the installation process is completed successfully, signal to the RingCentral desktop app that the install dialog can now be closed using the Javascript below, which toggles the enabled state of the dialog's submit/finish button.
import { RingCentralNotificationIntegrationHelper } from 'ringcentral-notification-integration-helper'
const app = new RingCentralNotificationIntegrationHelper()
// Notify RingCentral app that the integration can submit (or not).
// RingCentral app can then toggle submit button in RingCentral app UI
app.send({
canSubmit: true // or false if can not submit
})
// Receive message from RingCentral app that user has already
// clicked submit button so integration can proceed to submit.
app.on('submit', async function someSubmitFunction (e) {
console.log(e.data.payload)
// do something like submit
const submitSuccess = await doSubmit()
return {
status: !!submitSuccess // true means submit success
// RingCentral app will close integration window
}
})
Transmitting messages directly or acting as a proxy
Depending upon your role as a developer building an add-in, you will either post a properly formed message to post to an incoming webhook URL, or you will act as a proxy that will convert a webhook into a properly formed message. Let's break this down to help you decide the best way to architect your add-in.
Installing the incoming webhook directly
Let's say you are the proprietor of the service called "Acme" and a user is installing an add-in for Acme. In this case, you can install the incoming webhook URL directly in your service, and when an event is triggered, you can compose a message associated with that event and post it directly to the incoming webhook URL.
Acting as a proxy
If on the other hand you are a third-party building an integration on behalf of Acme. In this case, your app will be required to instruct Acme to deliver events to your service. Then your service will effectively translate the received webhook into a properly formed message and finally transmit that message to RingCentral via the incoming webhook URL. In this architectural model your add-in acts as a proxy, receiving events on one hand, and forwarding a message to its final destination on the other.
If you are acting as a proxy, a key requirement for the target service, in this case Acme, is that it supports a public API which can be used to install a webhook integration.
Editing versus creating a webhook
As discussed above, a user within RingCentral's desktop app can both install a new add-in, as well as edit an existing one. When editing an existing webhook, the developer should present the end user (via the iframe) with a form to edit their subscription preferences.
For example, let's say you built an add-in for Github, and a user installed and configured your add-in to send a message whenever a pull request was received for the repository mycompany/my_repos
. Then later the the user wished to update the add-in to be triggered when a pull request was received or a commit was made. In this circumstance, it is recommended that when the iframe is loaded for the associated incoming webhook, the form you would render would already have the checkbox associated with "pull requests" selected. That way the user would only need to check the box associated with "commits" before saving their preferences.