RingCentral RingEX™ Developer Guide
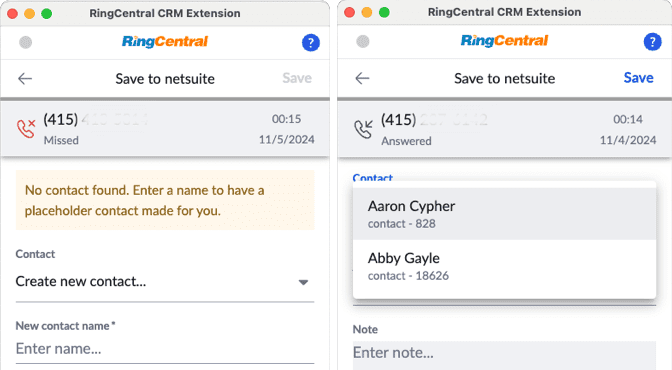
Embed a phone and log calls seamlessly in your CRM
RingCentral's App Connect, built on RingCentral Embeddable 2.0 is an all-in-one, complete, communication CRM integration solution. It embeds a fully-functional communications client into almost any app and helps users and admins to log all communications reliably in your app of choice. Developers can integrate with off-the-shelf SaaS products as well as proprietary CRMs using a simple developer framework.
Popular use cases
Send and receive SMS messages
Stay connected and engaged with customers at scale through messaging automation.
Use RingCentral to send and receive SMS, and access your SMS message history.
Synchronize call history
Download and process your entire company's call history for compliance and analytics.
Learn how to access your company's communication history:
Analyze call and meeting recordings
Archive and extract key data and insights from call recordings
RingCentral securely stores all media you generate and makes it available to you through an API.
Check out these popular developer topics
Authentication
Every app needs to authenticate to the RingCentral platform. Learn what auth method is best for your app.
Webhooks and events
Get notified when key events occur so that your app can respond to events in real-time.
Explore our APIs
Artificial intelligence
Generate transcripts and extract conversion insights from any media file.
Team messaging
Use RingCentral to post interactive messages so users can get more work done without leaving RingCentral.
Voice and telephony
Use RingCentral to enable a "click-to-dial" experience, and to manage calls in progress.
Meetings and video
Schedule meetings and access meeting history and recordings.
Analytics
Extract meaning insights into one's usage of the RingCentral communications platform.
Call handling
Manage active calls as they are happening in real-time
Getting Help
If on your way to building your first RingCentral application you encounter difficulty or need help, we are here to assist. Here are our most popular support resources available to you:
- Developer Forums - post a question to our support community.
- StackOverflow - seek help from one of the Internet's most popular Q&A sites for developers.
- Live Chat - post your question to our public Glip Team for live, real-time support during business hours.
Resources
API Reference
Consult our exhaustive API Reference Guide, and make API call using ZERO CODE.
Learn moreSDKs
We offer development libraries in a number of languages (C#, Java, Python, PHP, Javascript and more) to made building apps easier.
Learn moreAbout RingCentral
RingCentral is a leading provider of global enterprise cloud communications and collaboration solutions. More flexible and cost-effective than legacy on-premises systems, RingCentral empowers modern mobile and distributed workforces to communicate, collaborate, and connect from any location, on any device and via any mode. RingCentral provides unified voice, video, team messaging and collaboration, conferencing, online meetings, digital customer engagement and integrated contact center solutions for enterprises globally. RingCentral’s open platform integrates with leading business apps and enables customers to easily customize business workflows.